In a Redis Hashset(also known as HSet), data is organized into a mapping between a single key and multiple field-value pairs. This structure is particularly useful when representing entities with numerous attributes or properties. Leveraging the Hashset, developers can achieve not only efficient storage and retrieval of data but also logical grouping and manipulation of related information.
This introduction delves into the fundamentals of Redis Hashsets, exploring their syntax, use cases, and the advantages they bring to the table in terms of data modeling and retrieval efficiency. Whether you are new to Redis or seeking to optimize your data organization strategies, understanding the intricacies of the Hashset will undoubtedly enhance your ability to harness the full potential of Redis in diverse applications.
What is Redis Hashset?
A Redis Hash Set is a data structure that stores fields and values, where each field is associated with a value. It’s similar to a Python dictionary or a JavaScript object, making it ideal for representing structured data. You can think of a Hash as a collection of key-value pairs within Redis.
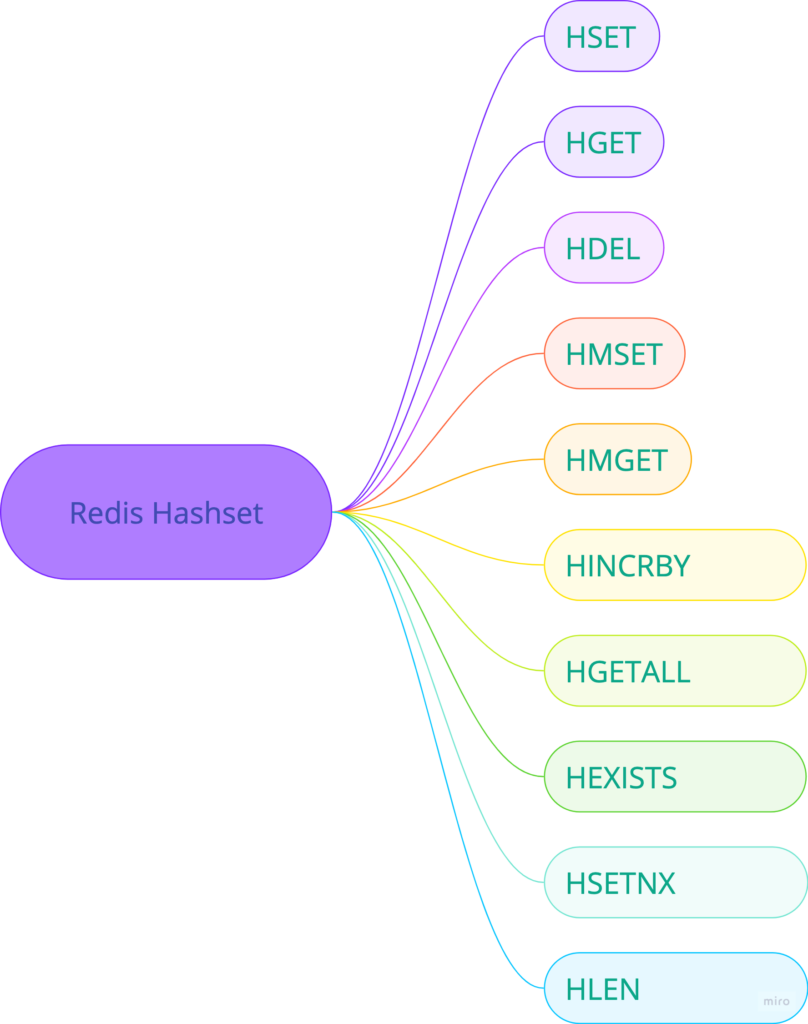
How Does Redis Hashset Work?
Under the hood, Redis implements Hash Sets as an efficient and compact data structure. The implementation ensures that Hash Sets perform well, even with many fields and values. Redis uses an open-addressing hash table, where multiple fields and values can be stored efficiently in a single key.
Use Cases for Redis Hashset
Hash Sets are versatile and can be used in various scenarios, including:
- Storing object-like data: Hash Sets are perfect for storing structured data like user profiles, product information, or configuration settings.
- Real-time analytics: You can use Hash Sets to capture and store event data, with fields representing different event properties.
- Caching complex data: Instead of serializing and deserializing complex data structures, you can store them as Hash Sets for efficient caching.
- Incrementing and counting: Hash Sets are great for tracking counts and performing increments, making them suitable for building features like counters and statistics.
Working with Redis Hashset
Let’s explore some basic commands and examples of how you would use Hash Sets in Redis.
Adding Fields and Values
To add a field and value to a Hash Set, you use the HSET
command:
# login to redis shell, e.g - redis-cli (or customise it for different port setting)
HSET user:1 username "john_doe"
HSET user:1 email "john@example.com"
This command creates a Hash Set with the key “user:1” and adds fields for the username and email.
Retrieving Fields and Values
To get a field’s value from a Hash Set, you can use the HGET
command:
HGET user:1 username
This command retrieves the username of “john_doe.”
Updating Fields and Values
To update the value of a field, use HSET
again with the same field name:
HSET user:1 username "new_username"
This updates the username field to “new_username.”
Deleting Fields
To remove a field and its value, use the HDEL
command:
HDEL user:1 email
This deletes the email field from the Hash Set.
Practical Example: User Profile
Let’s say you’re building a user management system. You can use a Hash Set to represent a user profile. Here’s how it might look:
HSET user:1 username "john_doe"
HSET user:1 email "john@example.com"
HSET user:1 age 30
# use HSETNX if you want to set only if it is not already set
HSETNX user:2 username "tom"
# get username
HGET user:1 username
# get username and email
HMGET user:1 username email
# get all field and values
HGETALL user:1
# delete age field
HDEL user:1 age
# check username field exists for user:1
HEXISTS user:1 username
# get number of fields in user:1
HLEN user:1
Now you have a structured user profile that’s easy to update and retrieve.
Redis hashset in go
Working with redis in Go is simple. See below the sample code for the main redis hashset operations.
package main
import (
"fmt"
"github.com/gomodule/redigo/redis"
)
func main() {
conn, err := redis.Dial("tcp", "localhost:6379")
if err != nil {
panic(err)
}
defer conn.Close()
// Create a new hash set named "myHashSet"
conn.Do("HSETNX", "myHashSet", "field1", "value1")
conn.Do("HSETNX", "myHashSet", "field2", "value2")
// Add more fields to the existing hash set
conn.Do("HSET", "myHashSet", "field3", "value3")
// Retrieve all fields and values from the hash set
values, err := redis.Values(conn.Do("HGETALL", "myHashSet"))
if err != nil {
panic(err)
}
for i := 0; i < len(values); i += 2 {
fmt.Println(string(values[i]), string(values[i+1]))
}
// Check if a field exists in the hash set
exists, err := redis.Bool(conn.Do("HEXISTS", "myHashSet", "field2"))
if err != nil {
panic(err)
}
fmt.Println("field2 exists:", exists)
// Remove a field from the hash set
conn.Do("HDEL", "myHashSet", "field1")
// Get the number of fields in the hash set
count, err := redis.Int(conn.Do("HLEN", "myHashSet"))
if err != nil {
panic(err)
}
fmt.Println("Number of fields:", count)
}
Top 10 FAQs on Redis HashSets
1. What are Redis HashSets and how do they work?
HashSets are data structures in Redis that store key-value pairs, similar to dictionaries or maps in other languages. They offer efficient retrieval and manipulation of related fields under a single key, unlike sorted sets or lists.
2. What are the benefits of using HashSets in Redis?
- Efficient key-value access: Retrieve specific fields quickly using their names.
- Group related data: Store diverse data types like strings, numbers, or nested Hashes under one key.
- Scalability: Handle large datasets efficiently as Hashes scale well with the number of fields.
- Atomicity: Operations like HSETNX or HDEL occur atomically, ensuring data consistency.
3. What are some common operations on HashSets?
- HSET/HMSET: Set one or multiple key-value pairs in the Hash.
- HGET/HMGET: Retrieve one or multiple values from the Hash.
- HEXISTS: Check if a specific field exists in the Hash.
- HDEL: Remove one or more fields from the Hash.
- HLEN: Get the number of fields in the Hash.
4. What are some use cases for HashSets in Redis?
- Storing user profiles with various attributes.
- Caching frequently accessed data with different fields.
- Implementing shopping cart functionality with items and their details.
- Representing complex objects with nested Hashes for structured data.
- Implementing session management with user data under a unique session key.
5. Are there any limitations to using HashSets?
- Limited sorting capabilities compared to sorted sets.
- Not ideal for large objects due to potential memory overhead.
- Not suitable for frequent value updates due to performance implications.
6. What are some best practices for using HashSets?
- Choose field names wisely for efficient lookups.
- Consider using HMSET/HMGET for bulk operations if applicable.
- Be mindful of potential memory usage for large Hashes.
- Implement expiration times if data has limited validity.
7. How do HashSets differ from other Redis data structures like Hashes and Lists?
- Regular Hashes offer similar key-value pairs but lack field names and atomic operations.
- Lists are ordered collections, good for sequences or FIFO data, unlike Hashes with unordered fields.
8. What are some security considerations when using HashSets?
- Securely manage sensitive data stored in Hashes with access control mechanisms.
- Consider encryption or hashing sensitive values before storing them.
- Regularly monitor and audit access to critical Hashes for security breaches.
9. Where can I find more resources and examples on Redis HashSets?
- Official Redis hash documentation
- Examples in your chosen Redis client library documentation.
10. When should I consider alternatives to HashSets in Redis?
- If you need ordered data, Sorted Sets might be a better choice.
- For simple key-value pairs without field names, regular Hashes could suffice.
- For frequently updated data with short lifespans, consider caching mechanisms outside Redis.
Conclusion
Redis Hashsets are a powerful tool for working with structured data, making them a valuable addition to your Redis toolkit. They’re fast, efficient, and flexible, making them suitable for a wide range of use cases, from user profiles to real-time analytics. By understanding how to work with Hash Sets, you can harness the full potential of Redis for handling structured data in your applications.
Happy coding!
2 thoughts on “Redis Hashset(HSet): A Master Guide with simple examples in 2024”