By applying Redis Sorted Sets in Go, you will drastically improve your user experience, and app performance and ultimately benefiting the business.
This post will delve into how to use Redis Sorted Sets in Go, how they work, and provide practical examples of their use. Find areas in your code where you can apply this to offload such responsibility to redis sorted sets.
Prerequisites:
- Learn about Redis Sorted Sets
- Setup redis. Refer to this post in case you need it.
What Are Redis Sorted Sets?
Sorted Sets are a data structure that, like Sets, are a collection of strings. What sets them apart is two key characteristics:
- Every member of a Sorted Set is unique. No two members can be identical, which makes them similar to the standard Set.
- Each member in a Sorted Set is associated with a score. This score is used to order the members from the lowest to the highest score. Members with the same score are then ordered lexicographically.
This dual nature of Sorted Sets allows them to handle a range of tasks that require both uniqueness and ordering.
How Do Sorted Sets in Redis Work?
Under the hood, Redis implements Sorted Sets using a data structure known as a skip list. Skip lists are ordered linked list that allows for O(logN) search time complexity, making them incredibly efficient for operations that require sorted data.
Use Cases for Sorted Sets in Redis
Sorted Sets are particularly useful for:
- Leaderboards and scoring: You can quickly update and retrieve player rankings.
- Time-series data: Sorted Sets can efficiently handle data where the key is a timestamp.
- Session store: Store user sessions with an expiration time as the score for easy expiration management.
- Autocomplete features: By leveraging the lexicographical ordering, you can implement autocomplete systems.
Working with Sorted Sets in Redis
Let’s go through some basic commands and examples of how you would use Sorted Sets in Redis.
Adding Members
To add a member to a Sorted Set, you use the ZADD
command:
ZADD myzset 1 "one"
This command adds the member “one” with a score of 1 to the myzset
Sorted Set.
Retrieving Members
To get members from a Sorted Set, you can use several commands, such as ZRANGE
:
ZRANGE myzset 0 -1 WITHSCORES
This command retrieves all members from myzset
along with their scores.
Updating Scores
To update the score of a member, you simply use ZADD
with the new score:
ZADD myzset 2 "one"
This updates the score of member “one” to 2 in myzset
.
Removing Members
To remove a member, use the ZREM
command:
ZREM myzset "one"
This removes the member “one” from myzset
.
Range Queries
Sorted Sets support range queries by score or by rank. For example:
ZRANGEBYSCORE myzset 1 3
This would return all members with scores between 1 and 3.
Practical Example: Implementing a Leaderboard
Imagine you’re building a gaming platform and need to maintain a leaderboard. Here’s how you might do that with a Sorted Set:
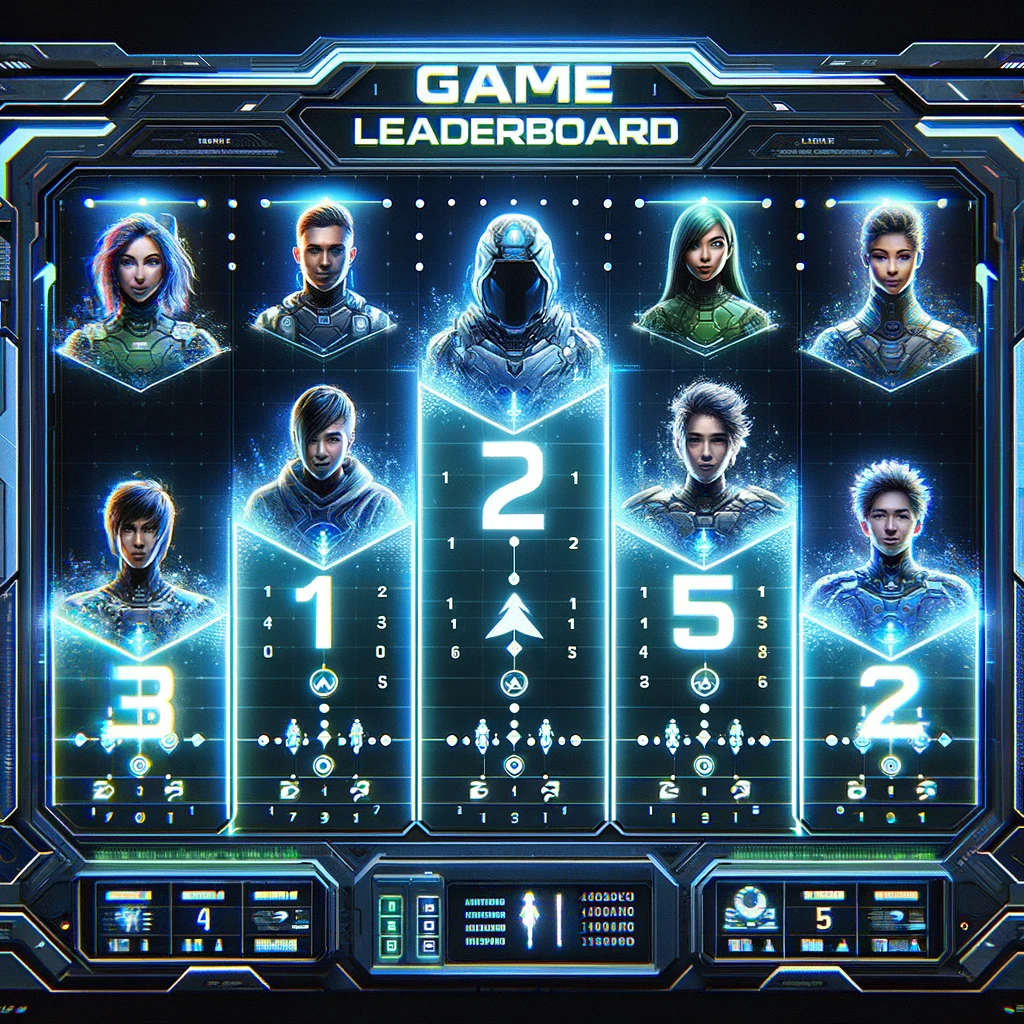
Add player scores:
ZADD leaderboard 5000 "player1"
ZADD leaderboard 7500 "player2"
Retrieve the top 3 players:
ZREVRANGE leaderboard 0 2 WITHSCORES
Update a player’s score:
ZINCRBY leaderboard 300 "player1"
Find a player’s rank:
ZRANK leaderboard "player1"
How to use Redis Sorted Sets in Go
See below the sample program in Go for the above use case
package main
import (
"fmt"
"github.com/go-redis/redis/v8"
"context"
)
func main() {
// Connect to Redis
rdb := redis.NewClient(&redis.Options{
Addr: "localhost:6379", // Redis server address
Password: "", // No password
DB: 0, // Default DB
})
defer rdb.Close()
ctx := context.Background()
// Add elements to the Sorted Set with scores
rdb.ZAdd(ctx, "leaderboard", &redis.Z{Score: 100, Member: "player1"})
rdb.ZAdd(ctx, "leaderboard", &redis.Z{Score: 200, Member: "player2"})
rdb.ZAdd(ctx, "leaderboard", &redis.Z{Score: 50, Member: "player3"})
// Get the rank of a player
rank, err := rdb.ZRevRank(ctx, "leaderboard", "player1").Result()
if err != nil {
fmt.Println("Error:", err)
return
}
fmt.Println("Player1's rank:", rank+1) // Rank starts from 0, so add 1
// Get the top players
topPlayers, err := rdb.ZRevRangeByScore(ctx, "leaderboard", &redis.ZRangeBy{
Min: "-inf",
Max: "+inf",
Offset: 0,
Count: 2, // Get top 2 players
}).Result()
if err != nil {
fmt.Println("Error:", err)
return
}
fmt.Println("Top players:", topPlayers)
}
Pay attention to how redis sorted sets is being used.
Similary you can apply in your projects.
Conclusion
Redis Sorted Sets offer an exceptional blend of performance and functionality for scenarios where you need ordered, unique elements. By understanding and leveraging this powerful data structure, you can build efficient, real-time applications that require sorted data, from leaderboards to scheduling systems.
Remember, Redis commands are quite intuitive, and with a little practice, you’ll be able to master Sorted Sets and the other data structures that Redis offers. Happy coding!
2 thoughts on “How to use Redis Sorted Sets in Go: A Simple Easy Guide in 20 Minutes”