Introduction
Working with JSON in Golang is a common and essential task for many developers. Go’s standard library provides the encoding/json
package to marshal Go data structures into JSON and vice versa. However, issues can arise when exporting fields and attempting to marshal or unmarshal data.
This post delves into the complexities surrounding exported fields in Go’s struct types and their impact on JSON marshaling. We will explore how field naming, struct tags, and the importance of exported fields can affect the serialization and deserialization process.
By the end of this article, you’ll have a clear understanding of how to handle JSON marshal issues stemming from exported fields, ensuring smooth data interchange in your Go applications.
Prerequisites:
- Install go by following this guide.
Understanding Marshaling/Unmarshaling of JSON in Golang
Before diving into JSON marshal issues, it’s crucial to comprehend how JSON marshaling works in Go. JSON (JavaScript Object Notation) is a lightweight data interchange format used to represent structured data. Go’s encoding/json
package allows us to encode Go data structures into JSON format and decode JSON into Go data structures.
In Go, the fundamental rule is that fields in a struct must be exported (i.e., their names start with an uppercase letter) to be included in the JSON marshaling process. Let’s start by examining the basics.
Consider a simple Person
struct:
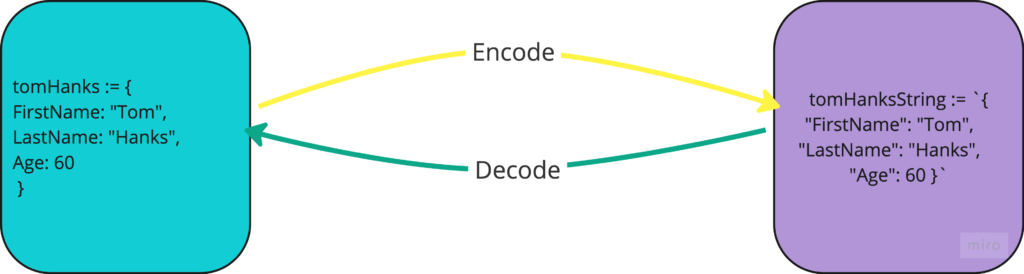
type Person struct {
FirstName string
LastName string
Age int
}
If you marshal an instance of this struct to JSON in Golang, it will look like this:
{
"FirstName": "Tom",
"LastName": "Hanks",
"Age": 60
}
Here, the exported fields FirstName
, LastName
, and Age
are serialized into JSON fields with the same names.
Field Naming of JSON in Go
By default, the JSON field names correspond to the struct field names. However, there may be scenarios where you want to specify custom field names in the JSON representation. You can achieve this by using struct tags.
Struct tags are metadata attached to struct fields that provide additional information to the encoder or decoder. In the context of JSON marshaling, you can use struct tags to specify the JSON field name. Here’s how you can modify the Person
struct:
// tag example of JSON in Go
type Person struct {
FirstName string `json:"first_name"`
LastName string `json:"last_name"`
Age int `json:"age"`
}
Now, when you marshal an instance of Person
, the JSON will have customized field names:
{
"first_name": "Tom",
"last_name": "Hanks",
"age": 60
}
Exported Fields and JSON Marshaling in Golang
Exported fields are vital for JSON marshaling in Go. If a field is not exported (i.e., its name starts with a lowercase letter), it won’t be considered during JSON encoding. Let’s illustrate this with an example:
type person struct {
firstName string
lastName string
age int
}
If you attempt to marshal an instance of this unexported person
struct, you will encounter issues:
p := person{"Tom", "Hanks", 60}
jsonData, err := json.Marshal(p)
if err != nil {
fmt.Println(err)
}
fmt.Println(string(jsonData))
This code will result in an empty JSON object:
{}
To fix this issue, you must export the fields by capitalizing their initial letters:
type Person struct {
FirstName string
LastName string
Age int
}
Handling Null Values
Another consideration when dealing with marshaling JSON in golang is how to handle null values. Null values are common in JSON data, but Go has a strong type system that requires variables to have non-nil values. This mismatch can lead to challenges when unmarshaling JSON that may contain null values in Go data structures.
The encoding/json
package provides a solution using pointers. If a struct field is a pointer type, it can be assigned a null value in the JSON data. Here’s an example:
type Person struct {
FirstName *string `json:"first_name"`
LastName *string `json:"last_name"`
Age *int `json:"age"`
}
With this structure, you can unmarshal JSON in Golang that contains null values:
{
"first_name": null,
"last_name": null,
"age": null
}
Circular References and Infinite Loops of JSON in Golang
JSON encoding and decoding in Go are not immune to circular references in your data structures. Circular references occur when multiple objects reference each other in a loop, potentially causing infinite loops during encoding or decoding. Go’s encoding/json
package does not handle circular references well by default.
Consider the following example:
type Person struct {
FirstName string
Friends []*Person
}
john := &Person{
FirstName: "John",
}
jane := &Person{
FirstName: "Jane",
Friends: []*Person{john},
}
john.Friends = append(john.Friends, jane)
If you attempt to marshal the jane
object, the encoding/json
package will encounter an infinite loop when trying to marshal jane
‘s friends, who in turn reference jane
. To avoid this, you can use struct tags to indicate which fields should be included in the JSON in Go encoding:
type Person struct {
FirstName string `json:"first_name"`
Friends []*Person `json:"-"`
}
With this json:"-"
, you can exclude the Friends
field from JSON encoding. This prevents infinite loops but may result in incomplete JSON data.
Advanced Custom Marshaling Unmarshaling
Sometimes, the default JSON encoding and decoding behavior is insufficient for complex data structures. In such cases, you can implement custom marshaling and unmarshaling methods for your struct types.
Custom marshaling is achieved by implementing the MarshalJSON
method, which should return the JSON representation of the object. Conversely, custom unmarshaling is implemented by the UnmarshalJSON
method, which populates the object’s fields based on the provided JSON data.
Here’s an example:
type Person struct {
FirstName string `json:"first_name"`
LastName string `json:"last_name"`
Age int `json:"age"`
}
// MarshalJSON will override default MarshalJSON, this provides a powerful way of custom marshalling in a simple way
func (p Person) MarshalJSON() ([]byte, error) {
// Create a custom JSON object
customJSON := struct {
Name string `json:"full_name"`
Age int `json:"age"`
}{
Name: p.FirstName + " " + p.LastName,
Age: p.Age,
}
// Marshal the custom JSON object
return json.Marshal(customJSON)
}
// UnmarshalJSON will override default UnmarshalJSON, this provides a powerful way of custom unmarshalling in a simple way
func (p *Person) UnmarshalJSON(data []byte) error {
// Create a custom JSON object to decode into
var customJSON struct {
Name string `json:"full_name"`
Age int `json:"age"`
}
// Unmarshal the JSON data into the custom object
if err := json.Unmarshal(data, &customJSON); err != nil {
return err
}
// Split the full name into first and last name
names := strings.Fields(customJSON.Name)
if len(names) >= 2 {
p.FirstName = names[0]
p.LastName = names[1]
}
p.Age = customJSON.Age
return nil
}
// usage in client code
tom := Person{
FirstName: "Tom",
LastName: "Hanks",
Age: 60,
}
jsonData, err := json.Marshal(tom)
if err != nil {
fmt.Println("JSON marshaling error:", err)
return
}
fmt.Println(string(jsonData))
// unmarshall operation
var newTom Person
err = json.Unmarshall(jsonData, &newTom)
fmt.Println(newTom)
In this advanced custom marshaling example, we’ve implemented the MarshalJSON
and UnmarshalJSON
methods for the Person
struct.
- In the
MarshalJSON
method, we create a custom JSON structure with the fields"full_name"
and"age"
. We populate this structure based on thePerson
struct’s fields and then marshal the custom JSON structure. - In the
UnmarshalJSON
method, we define a custom JSON structure and unmarshal the provided JSON data into it. We extract the full name and age from the custom structure and assign the values to the corresponding fields in thePerson
struct.
Custom marshaling and unmarshaling JSON in Go allow you to have fine-grained control over how your Go types are encoded and decoded to and from JSON. This can be particularly useful when working with complex or non-standard JSON representations. This flexibility is precious when dealing with APIs or data sources that use non-standard JSON formats or when you need to transform data during encoding and decoding.
10 FAQs on JSON in Golang
1. What is the encoding/json package in Go?
This package provides functions for encoding and decoding JSON data in Go. You can use it to convert Go structs to JSON format and vice versa.
2. How do I encode a Go struct to JSON?
Use the Marshal
function from the encoding/json
package.
type User struct {
Name string `json:"name"`
Age int `json:"age"`
}
user := User{"John Doe", 30}
data, err := json.Marshal(user)
// Check for errors
fmt.Println(string(data)) // Output: {"name":"John Doe","age":30}
3. How do I decode JSON data into a Go struct?
Use the Unmarshal
function from the encoding/json
package.
var user User
data := []byte(`{"name":"Jane Doe","age":25}`)
err := json.Unmarshal(data, &user)
// Check for errors
fmt.Println(user.Name, user.Age) // Output: Jane Doe 25
4. Can I customize how JSON is encoded/decoded?
Yes! You can use struct tags to control the field names, and format, and even include/exclude fields during encoding/decoding.
5. How do I deal with complex data structures like nested objects and arrays?
Nested objects and arrays are handled seamlessly by encoding/json. Structs with nested structs and slices represent them in the JSON output.
6. How do I validate JSON data before decoding it?
For basic validation, you can use the json.Valid
function to check if the JSON data is syntactically correct. For more complex validation, use third-party packages like go-playground/validator
.
7. What are some best practices for using JSON in Golang?
- Use meaningful struct tag names for clarity.
- Validate JSON data before decoding to avoid errors.
- Consider using custom encoders/decoders for complex data types.
- Prefer readable formats over minified JSON when sharing data.
8. Are there any alternatives to the encoding/json package?
Yes, libraries like fastjson
and gjson
offer different performance and feature sets. However, encoding/json
is the standard library option and generally recommended for most use cases.
9. How does working with JSON in Golang compare to other languages like Python or JavaScript?
Go’s approach emphasizes static typing and explicit struct definitions, while languages like Python or JavaScript are more dynamic. It offers stronger type safety and predictability but might require more upfront work compared to dynamic approaches.
10. How to do Custom Marshaling Unmarshaling of JSON in Golang?
Please read through the Custom Marshaling Unmarshaling section in this post and practice.
Conclusion
In the world of Go and JSON data interchange, understanding the intricacies of JSON marshaling, and how exported fields impact this process, is crucial. We’ve explored the fundamental concepts of JSON marshaling in Go, including the role of exported fields, struct tags, and handling null values.
The significance of exported fields cannot be overstated. Fields must be exported (begin with an uppercase letter) to be considered during JSON encoding. Struct tags allow you to customize field names in the JSON representation, providing control over how your data appears in the JSON output.
Handling null values is also a vital consideration. Using pointers for fields in your struct allows you to accurately represent null values in JSON data.
Furthermore, we’ve discussed advanced techniques, including custom JSON marshaling and unmarshaling. Implementing the MarshalJSON
and UnmarshalJSON
methods provide the ability to tailor the JSON encoding and decoding process to your specific needs.
By mastering these JSON marshaling techniques and understanding the role of exported fields, you can seamlessly exchange data between Go applications and external systems, ensuring that your data is accurately represented and your code remains flexible and maintainable. This knowledge empowers you to navigate the complexities of working with JSON in Go with confidence, no matter the challenges you encounter.