Introduction
Error in Go is similar to exception in other languages but it is explicit and better. An error is just a value in go like any other value in go
A. Significance of Effective Error Handling in Go
Effective error handling is a critical aspect of writing robust and reliable software. It ensures that a program can gracefully handle unexpected situations, preventing crashes and providing meaningful feedback to users or developers. By anticipating and managing errors, developers can enhance their applications’ overall stability and user experience.
B. Go’s Unique Approach to Error Handling
Go, also known as Golang, adopts a distinctive approach to error handling that sets it apart from many other programming languages. Instead of relying on exceptions, Go encourages the use of explicit error values. This design choice promotes cleaner and more predictable code, as errors are treated as regular values that can be inspected and managed explicitly. This approach aligns with Go’s philosophy of simplicity and readability, contributing to code that is less prone to unexpected behaviors.
C. The Use of Explicit Error Values in Error Handling
In Go, functions that may encounter errors return multiple values, typically including an error as one of the return values. Developers are encouraged to check the error value explicitly using a if
statement to determine if the operation was successful. This transparency simplifies the flow of control, making it clear when and where errors occur. Additionally, the use of named return values in Go functions contributes to more expressive and self-documenting code, aiding in the understanding of error-handling logic.
Prerequisites
- Learn the basics of Go
- Install Go
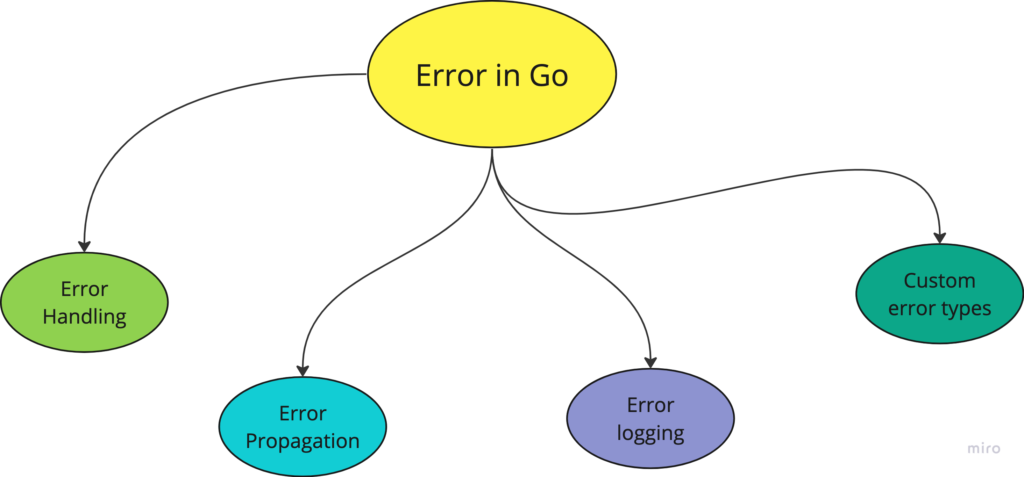
Error Types in Go
A. Standard Errors and the Error Interface
In Go, errors are treated as values and the built-in error
interface plays a pivotal role in error handling. The error
interface is simple yet powerful, consisting of a single method:
type error interface {
Error() string
}
Any type that implements this interface is considered an error in Go. Standard errors in the language are often represented by the errors
package, which provides a convenient New
function for creating basic errors:
Developers can then use the Error()
method to retrieve the error message and handle errors accordingly.
B. Custom Errors and Error Types
While standard errors are suitable for many scenarios, Go allows developers to define custom error types by implementing the error
interface in their own types. This flexibility enables the creation of richer error information, including additional context or metadata. For example:
type CustomError struct {
Code int
Message string
}
func (e CustomError) Error() string {
return fmt.Sprintf("Error %d: %s", e.Code, e.Message)
}
By incorporating custom error types, developers can create more expressive error messages and differentiate between various error conditions within their applications. This promotes clarity in error handling and allows for more nuanced responses to different error scenarios.
Understanding the interplay between standard errors, the error
interface, and custom error types is essential for effective error management in Go. It empowers developers to craft informative and actionable error messages that contribute to the overall reliability and maintainability of their code.
C. Error Composition and Wrapping
In Go, error composition and wrapping provide powerful mechanisms for adding context and additional information to errors, facilitating better error tracking and debugging.
Error Composition:
Error composition involves combining multiple errors into a single error value. This is achieved using the fmt.Errorf
function, which formats an error message and can embed other errors within it:
package main
import (
"fmt"
"errors"
)
func exampleFunction() error {
innerError := errors.New("An inner error")
return fmt.Errorf("Outer error: %w", innerError)
}
The %w
verb in fmt.Errorf
indicates that the following error should be wrapped within the new error. This composition maintains the original error information while providing a higher-level context.
Error Wrapping:
Error wrapping, introduced in Go 1.13 with the errors
package, allows developers to annotate errors with additional context. The errors.Wrap
function creates a new error that wraps an existing error, providing a more detailed description:
package main
import (
"fmt"
"github.com/pkg/errors"
)
func exampleFunction() error {
innerError := errors.New("An inner error")
return errors.Wrap(innerError, "Encountered an issue")
}
With error wrapping, the resulting error not only includes the original error message but also a new message that adds context to the error’s origin.
func main() {
err := exampleFunction()
if err != nil {
fmt.Printf("Error: %v\n", err)
}
}
Output
Error: Encountered an issue: An inner error
By embracing error composition and wrapping, Go developers can build a hierarchical structure of errors that not only conveys the nature of the problem but also aids in understanding the sequence of events leading to the error. This practice enhances the troubleshooting process and fosters more effective error management in Go applications.
Return Values and Errors in Go
A. Using Multiple Return Values for Errors
One of the distinctive features of Go is its support for multiple return values. This feature is particularly powerful in the context of error handling. Functions in Go often return both a result and an error, allowing the caller to easily check for success or failure:
package main
import "fmt"
// divide returns the result of a division and an error if the divisor is zero.
func divide(dividend, divisor float64) (float64, error) {
if divisor == 0 {
return 0, fmt.Errorf("division by zero")
}
result := dividend / divisor
return result, nil
}
func main() {
result, err := divide(10, 2)
if err != nil {
fmt.Printf("Error: %v\n", err)
} else {
fmt.Printf("Result: %f\n", result)
}
}
In this example, the divide
function returns both the result of the division and an error if the divisor is zero. The caller can easily inspect the error value to handle exceptional cases.
B. Common Practice of Returning (Result, Error)
It has become a common practice in Go to return a pair of values: the result of the operation and an error. This practice simplifies error handling and encourages developers to check for errors explicitly. The signature of such functions typically looks like this:
func exampleFunction() (resultType, error) {
// Implementation...
if err != nil {
return nil, err
}
return result, nil
}
This pattern allows for a clear and consistent approach to error handling across Go codebases. Developers can easily understand and reason about functions that adhere to this convention.
By leveraging multiple return values for errors, Go enables concise and expressive error handling, promoting the creation of robust and readable code. Developers are encouraged to embrace this pattern for clearer and more effective error management in their Go applications.
C. Checking Errors with if err != nil
Idiom (core of error handling)
In Go, the if err != nil
idiom is a fundamental and pervasive approach to checking errors. Since Go encourages the explicit handling of errors, this idiom is widely adopted across codebases to ensure robust error management.
package main
import (
"fmt"
"os"
)
func readFile(filePath string) ([]byte, error) {
file, err := os.Open(filePath)
if err != nil {
return nil, err
}
defer file.Close()
data := make([]byte, 1024)
_, err = file.Read(data)
if err != nil {
return nil, err
}
return data, nil
}
func main() {
filePath := "example.txt"
data, err := readFile(filePath)
if err != nil {
fmt.Printf("Error reading file %s: %v\n", filePath, err)
return
}
fmt.Printf("File content: %s\n", data)
}
In this example, the readFile
function attempts to open a file, read its content, and return the data. At each step where an operation could potentially fail, the function checks for an error using if err != nil
. If an error occurs, it is returned immediately, allowing the caller to handle the error appropriately.
The main
function then calls readFile
and checks the returned error. If an error is present, it prints an error message; otherwise, it proceeds to use the file data.
This idiom ensures that errors are explicitly acknowledged and handled at the point of occurrence, promoting code clarity and reducing the likelihood of unnoticed errors. The if err != nil
construct serves as a visual cue for developers to address error conditions diligently, contributing to the overall reliability of Go programs.
Error Propagation in Go
A. Propagating Errors Up the Call Stack
In Go, error propagation involves allowing errors to propagate up the call stack, reaching higher-level functions or even the main program for handling. This mechanism ensures that errors are not silently ignored but are addressed at the appropriate level.
package main
import (
"fmt"
"os"
)
func readFile(filePath string) ([]byte, error) {
file, err := os.Open(filePath)
if err != nil {
return nil, fmt.Errorf("readFile: %w", err)
}
defer file.Close()
data := make([]byte, 1024)
_, err = file.Read(data)
if err != nil {
return nil, fmt.Errorf("readFile: %w", err)
}
return data, nil
}
func processFile(filePath string) error {
data, err := readFile(filePath)
if err != nil {
return fmt.Errorf("processFile: %w", err)
}
// Process the file data...
return nil
}
func main() {
filePath := "example.txt"
err := processFile(filePath)
if err != nil {
fmt.Printf("Error processing file %s: %v\n", filePath, err)
}
}
In this example, the readFile
function propagates errors using the %w
verb with fmt.Errorf
. This way, the calling function, processFile
, can recognize the specific origin of the error. The error is then further propagated up to the main
function, where it is finally handled.
B. Error Handling at the Appropriate Level
Each function along the call stack is responsible for handling errors relevant to its context. By allowing errors to propagate, the program achieves a separation of concerns, with each function focusing on its specific responsibilities.
func processFile(filePath string) error {
data, err := readFile(filePath)
if err != nil {
return fmt.Errorf("processFile: %w", err)
}
// Process the file data...
return nil
}
In the processFile
function, if an error occurs during file reading, it is wrapped with additional context and returned. This ensures that error information retains its specificity as it moves up the call stack.
C. Techniques for Handling Errors Gracefully
- Logging:
- Log errors at the point of occurrence using a logging library like
log
or a structured logger. This provides valuable information for debugging.
- Log errors at the point of occurrence using a logging library like
func readFile(filePath string) ([]byte, error) {
file, err := os.Open(filePath)
if err != nil {
log.Printf("Error opening file %s: %v", filePath, err)
return nil, fmt.Errorf("readFile: %w", err)
}
// ...
}
Wrap and Annotate:
- Use
fmt.Errorf
to wrap errors with additional context, preserving the original error information.
func readFile(filePath string) ([]byte, error) {
// ...
if err != nil {
return nil, fmt.Errorf("readFile: %w", err)
}
// ...
}
Returning Specific Errors:
- Define custom error types to convey specific information about error conditions, making it easier to handle them at higher levels.
type FileNotFoundError struct {
FilePath string
}
func (e FileNotFoundError) Error() string {
return fmt.Sprintf("File not found: %s", e.FilePath)
}
func readFile(filePath string) ([]byte, error) {
// ...
if os.IsNotExist(err) {
return nil, FileNotFoundError{FilePath: filePath}
}
// ...
}
By adopting these techniques, developers can propagate errors effectively, handle them at the appropriate level, and enhance error messages with useful context, contributing to more resilient and maintainable Go code.
Defer and Error Handling
A. Leveraging defer
for Cleanup Actions
In Go, the defer
statement allows developers to schedule a function call to be executed just before a function returns. This feature is particularly valuable for handling cleanup tasks, and ensuring resources are released, regardless of whether an error occurs during the function’s execution.
package main
import (
"fmt"
"os"
)
func processFile(filePath string) error {
file, err := os.Open(filePath)
if err != nil {
return fmt.Errorf("error opening file: %w", err)
}
defer file.Close() // Ensures file is closed even if an error occurs
// Further processing...
return nil
}
In this example, defer file.Close()
guarantees that the Close
function will be called before processFile
exits, irrespective of whether an error occurs or not. This helps prevent resource leaks and ensures proper cleanup.
Error Logging
A. Logging Errors for Debugging and Monitoring
In the realm of Go programming, effective error logging is pivotal for debugging, monitoring, and maintaining the health of applications. Properly logging errors provides valuable insights into the runtime behavior, aiding developers in identifying issues and ensuring smoother operations.
package main
import (
"fmt"
"log"
"os"
)
func processFile(filePath string) error {
file, err := os.Open(filePath)
if err != nil {
log.Printf("Error opening file %s: %v", filePath, err)
return fmt.Errorf("error opening file: %w", err)
}
defer file.Close()
// Further processing...
return nil
}
In this example, the log.Printf
statement captures and prints the error along with additional context. This practice is instrumental during development and debugging phases.
B. Best Practices for Error Logging in Production
- Contextual Logging:
- Include relevant context information in error logs to aid in diagnosing issues.
func processRequest(req *http.Request) error {
// Processing logic...
if err != nil {
log.Printf("Error processing request %s: %v", req.URL.Path, err)
return fmt.Errorf("error processing request: %w", err)
}
return nil
}
Severity Levels:
- Use different severity levels (info, warning, error) for various log messages to prioritize and categorize issues.
func fetchData() error {
// Fetching data...
if err != nil {
log.Printf("Error fetching data: %v", err) // Error severity
return fmt.Errorf("error fetching data: %w", err)
}
return nil
}
C. Integrating Logging Libraries for Improved Error Visibility
- Logrus:
- Logrus is a popular logging library for Go, offering structured logging and various output formats.
package main
import (
"fmt"
log "github.com/sirupsen/logrus"
)
func main() {
log.SetFormatter(&log.TextFormatter{})
log.SetLevel(log.InfoLevel)
err := someFunction()
if err != nil {
log.WithFields(log.Fields{
"function": "someFunction",
"error": err,
}).Error("Error in someFunction")
}
}
Zap:
- Zap is a fast and structured logging library for Go, designed for high performance.
package main
import (
"fmt"
"go.uber.org/zap"
)
func main() {
logger, _ := zap.NewProduction()
defer logger.Sync()
err := someFunction()
if err != nil {
logger.Error("Error in someFunction", zap.Error(err))
}
}
Integrating dedicated logging libraries like Logrus or Zap provides advanced features such as structured logging, flexible output formats, and improved performance, enhancing error visibility in production environments.
By implementing robust error logging practices, developers can streamline the troubleshooting process, reduce downtime, and maintain the overall health of their Go applications.
Handling External Errors in Go
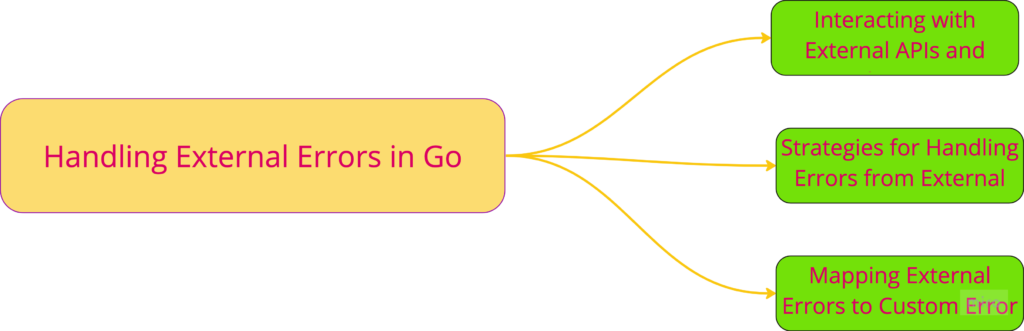
A. Interacting with External APIs and Libraries
Interacting with external APIs and libraries is a common task in Go development. Handling errors that originate from these external sources requires a thoughtful approach to ensure the robustness of your application.
package main
import (
"fmt"
"net/http"
)
func fetchDataFromAPI() ([]byte, error) {
resp, err := http.Get("https://api.example.com/data")
if err != nil {
return nil, fmt.Errorf("error fetching data from API: %w", err)
}
defer resp.Body.Close()
// Check the HTTP status code
if resp.StatusCode != http.StatusOK {
return nil, fmt.Errorf("unexpected status code: %d", resp.StatusCode)
}
// Read and return the data
data, err := ioutil.ReadAll(resp.Body)
if err != nil {
return nil, fmt.Errorf("error reading response body: %w", err)
}
return data, nil
}
In this example, the function fetchDataFromAPI
interacts with an external API. Any errors encountered during the HTTP request or response handling are wrapped to provide additional context.
B. Strategies for Handling Errors from External Sources
- Logging and Contextual Information:
- Log errors with contextual information to aid in debugging and troubleshooting.
func processResponse(resp *http.Response) error {
// Processing logic...
if resp.StatusCode != http.StatusOK {
return fmt.Errorf("unexpected status code: %d", resp.StatusCode)
}
return nil
}
Graceful Degradation:
- Implement graceful degradation strategies when interacting with external services. Provide fallback mechanisms or default values to handle temporary outages or unexpected responses.
func fetchDataFromAPI() (string, error) {
// Fetch data...
if err != nil {
return "default value", fmt.Errorf("error fetching data: %w", err)
}
return data, nil
}
C. Mapping External Errors to Custom Error Types
- Defining Custom Error Types:
- Create custom error types to represent specific error conditions arising from external sources.
type APIError struct {
StatusCode int
Message string
}
func (e APIError) Error() string {
return fmt.Sprintf("API error: %s (status code: %d)", e.Message, e.StatusCode)
}
Mapping External Errors:
- Map external errors to custom error types for more granular error handling.
func fetchDataFromAPI() ([]byte, error) {
resp, err := http.Get("https://api.example.com/data")
if err != nil {
return nil, fmt.Errorf("error fetching data from API: %w", err)
}
defer resp.Body.Close()
// Check the HTTP status code
if resp.StatusCode != http.StatusOK {
return nil, APIError{
StatusCode: resp.StatusCode,
Message: "Unexpected status code",
}
}
// Read and return the data
data, err := ioutil.ReadAll(resp.Body)
if err != nil {
return nil, fmt.Errorf("error reading response body: %w", err)
}
return data, nil
}
By mapping external errors to custom error types, developers can differentiate between various error conditions, allowing for more precise error handling and providing better insights into the nature of issues originating from external sources.
Handling external errors in Go involves a combination of logging, graceful degradation, and the creation of custom error types. These practices contribute to the resilience and reliability of applications interacting with external APIs and libraries.
Unit Testing and Error Handling in Go
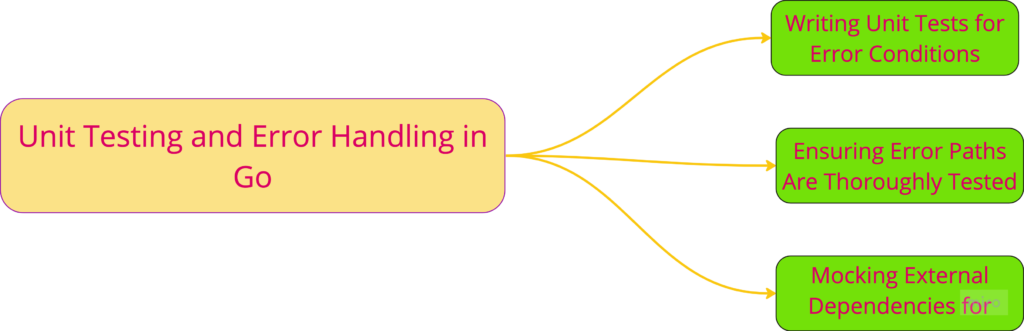
A. Writing Unit Tests for Error Conditions
Writing comprehensive unit tests for error scenarios is crucial to ensure the reliability and correctness of Go applications. By systematically testing error paths, developers can identify and address potential issues, enhancing the overall robustness of their code.
package main
import (
"errors"
"testing"
)
func Divide(a, b float64) (float64, error) {
if b == 0 {
return 0, errors.New("division by zero")
}
return a / b, nil
}
func TestDivide(t *testing.T) {
t.Run("Valid Division", func(t *testing.T) {
result, err := Divide(10, 2)
if err != nil {
t.Errorf("Unexpected error: %v", err)
}
if result != 5 {
t.Errorf("Expected result: 5, got: %f", result)
}
})
t.Run("Division by Zero", func(t *testing.T) {
_, err := Divide(10, 0)
if err == nil {
t.Error("Expected error, but got nil")
}
if err.Error() != "division by zero" {
t.Errorf("Unexpected error message: %v", err)
}
})
}
In this example, the Divide
function is tested with both valid and error-inducing scenarios. The TestDivide
function checks for the expected outcomes, ensuring that errors are correctly handled.
B. Ensuring Error Paths Are Thoroughly Tested
To achieve robust error handling, developers must diligently test various error paths within their code. This involves considering edge cases, boundary conditions, and scenarios where external dependencies may fail. Comprehensive testing helps uncover hidden issues and improves the overall stability of the application.
package main
import (
"errors"
"testing"
)
func ProcessData(data []int) error {
if len(data) == 0 {
return errors.New("empty data")
}
// Processing logic...
return nil
}
func TestProcessData(t *testing.T) {
t.Run("Valid Data", func(t *testing.T) {
err := ProcessData([]int{1, 2, 3})
if err != nil {
t.Errorf("Unexpected error: %v", err)
}
})
t.Run("Empty Data", func(t *testing.T) {
err := ProcessData([]int{})
if err == nil {
t.Error("Expected error, but got nil")
}
if err.Error() != "empty data" {
t.Errorf("Unexpected error message: %v", err)
}
})
}
The TestProcessData
function demonstrates testing for both valid and error scenarios, ensuring that the function correctly handles the case of empty data.
C. Mocking External Dependencies for Controlled Error Scenarios
Mocking external dependencies in unit tests allows developers to control the behavior of these dependencies, including inducing error conditions for thorough testing. Libraries like testify/mock
can be used to create mock objects that simulate external interactions.
package main
import (
"errors"
"testing"
"github.com/stretchr/testify/mock"
)
// ExternalService represents an external dependency.
type ExternalService interface {
PerformAction() error
}
// MockService is a mock implementation of ExternalService.
type MockService struct {
mock.Mock
}
func (m *MockService) PerformAction() error {
args := m.Called()
return args.Error(0)
}
func UseExternalService(service ExternalService) error {
return service.PerformAction()
}
func TestUseExternalService(t *testing.T) {
t.Run("Success", func(t *testing.T) {
mockService := new(MockService)
mockService.On("PerformAction").Return(nil)
err := UseExternalService(mockService)
if err != nil {
t.Errorf("Unexpected error: %v", err)
}
mockService.AssertExpectations(t)
})
t.Run("Failure", func(t *testing.T) {
mockService := new(MockService)
mockService.On("PerformAction").Return(errors.New("mock error"))
err := UseExternalService(mockService)
if err == nil {
t.Error("Expected error, but got nil")
}
if err.Error() != "mock error" {
t.Errorf("Unexpected error message: %v", err)
}
mockService.AssertExpectations(t)
})
}
In this example, the MockService
is used to simulate an external dependency. By controlling the behavior of the mock, developers can test how their code responds to various error conditions.
By incorporating these practices into the testing workflow, developers can confidently identify and address error-handling issues, resulting in more robust and reliable Go applications.
Best Practices for Error Handling in Go
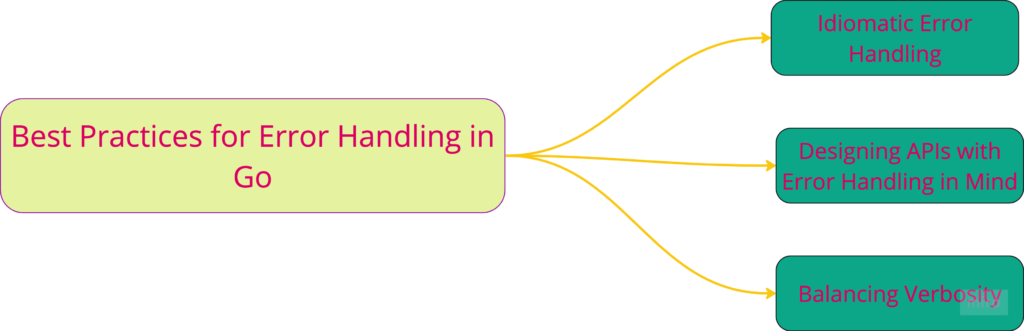
A. Idiomatic Error Handling in Go
Go promotes a distinctive and idiomatic approach to error handling that emphasizes simplicity, clarity, and explicitness. Understanding and following these principles enhances the readability and maintainability of Go code.
- Use of
error
Interface:- Go uses the
error
interface for representing errors. Functions often return anerror
as the last return value to indicate success or failure.
- Go uses the
func divide(a, b int) (int, error) {
if b == 0 {
return 0, errors.New("division by zero")
}
return a / b, nil
}
Error Wrapping with fmt.Errorf
:
- Utilize
fmt.Errorf
to wrap errors with additional context, providing more information for debugging.
func fetchDataFromAPI() ([]byte, error) {
resp, err := http.Get("https://api.example.com/data")
if err != nil {
return nil, fmt.Errorf("error fetching data from API: %w", err)
}
defer resp.Body.Close()
// Processing logic...
return data, nil
}
Checking for Specific Errors:
- Use type assertions or type switches to check for specific error types and handle them accordingly.
err := someFunction()
if netErr, ok := err.(net.Error); ok && netErr.Timeout() {
// Handle timeout error...
} else if err != nil {
// Handle other errors...
}
B. Designing APIs with Error Handling in Mind
- Return Explicit Error Values:
- Clearly define and document the error values that a function may return. This helps users of your API understand potential failure scenarios.
func processRequest(req *http.Request) (*http.Response, error) {
// Processing logic...
if err != nil {
return nil, fmt.Errorf("error processing request: %w", err)
}
return response, nil
}
Avoid Shadowing Errors:
- Avoid re-declaring variables with the same name in nested scopes, as it may lead to unintentional shadowing of error values.
func fetchData() error {
data, err := fetchFromDatabase()
if err != nil {
return fmt.Errorf("error fetching data: %w", err)
}
// Use data...
return nil
}
C. Balancing Verbosity and Clarity in Error Messages
- Include Relevant Context:
- Provide context information in error messages to facilitate debugging. Include details that help identify the root cause of the error.
func processFile(filePath string) error {
file, err := os.Open(filePath)
if err != nil {
return fmt.Errorf("error opening file %s: %w", filePath, err)
}
defer file.Close()
// Further processing...
return nil
}
Strive for Clarity:
- Aim for error messages that are clear and concise. Avoid unnecessary verbosity while ensuring that the message conveys essential information.
func fetchData() error {
resp, err := http.Get("https://api.example.com/data")
if err != nil {
return fmt.Errorf("failed to fetch data: %w", err)
}
defer resp.Body.Close()
// Processing logic...
return nil
}
By adhering to these best practices, developers can create error-handling mechanisms that align with Go’s idioms, design APIs that promote transparency, and strike a balance between providing sufficient information and maintaining clarity in error messages.
Real-World Examples and Use Cases in Go Error Handling
A. Examples from Popular Go Libraries
- net/http:
- The
net/http
package in the Go standard library provides robust error handling. For instance, thehttp.Get
function returns errors for various scenarios, allowing developers to handle network-related issues gracefully.
- The
resp, err := http.Get("https://www.example.com")
if err != nil {
log.Printf("HTTP request failed: %v", err)
// Handle error...
}
context:
- The
context
package is often used for cancellation and timeout handling. Errors returned from contexts provide valuable information about the reason for cancellation.
ctx, cancel := context.WithTimeout(context.Background(), 5*time.Second)
defer cancel()
result, err := someFunction(ctx)
if err != nil {
log.Printf("Operation canceled: %v", err)
// Handle cancellation...
}
B. Demonstrations of Effective Error Handling in Practical Scenarios
- File Operations:
- When working with file operations, the
os
package offers clear error handling. For instance, when opening a file, the code checks for errors and provides meaningful context.
- When working with file operations, the
file, err := os.Open("example.txt")
if err != nil {
log.Printf("Error opening file: %v", err)
// Handle error...
}
defer file.Close()
Database Interactions:
- Database interactions, as seen in popular libraries like
database/sql
, showcase effective error handling. Checking for errors during query execution is essential for identifying issues.
rows, err := db.Query("SELECT * FROM users")
if err != nil {
log.Printf("Error executing query: %v", err)
// Handle database error...
}
defer rows.Close()
C. Learning from Well-Established Go Projects
- Docker:
- The Docker project demonstrates effective error handling in a complex system. Codebase analysis reveals clear error messages and the use of context-specific error types.
func pullImage(imageName string) error {
// Docker pull logic...
if err != nil {
return fmt.Errorf("failed to pull image %s: %w", imageName, err)
}
// Further processing...
return nil
}
Kubernetes:
- Kubernetes, being a prominent Go project, exhibits comprehensive error handling. The codebase employs custom error types and provides detailed error messages for better debugging.
func createPod(podSpec *v1.Pod) error {
// Pod creation logic...
if err != nil {
return &PodCreationError{PodName: podSpec.Name, Reason: "Failed to create pod", Err: err}
}
// Further processing...
return nil
}
Studying real-world examples from popular Go libraries, practical scenarios, and established projects is invaluable for gaining insights into effective error handling practices. These examples showcase how to structure error messages, create custom error types, and handle errors in diverse contexts, contributing to the development of robust and maintainable Go applications.
Conclusion
A. Summary of Key Concepts on Error Handling in Go
In conclusion, mastering error handling in Go involves understanding and applying key concepts that contribute to the language’s simplicity, clarity, and reliability. The fundamental principles include:
- Idiomatic Approach:
- Embrace Go’s idiomatic error handling by using the
error
interface and returning explicit error values from functions.
- Embrace Go’s idiomatic error handling by using the
- Error Wrapping:
- Leverage
fmt.Errorf
to wrap errors with additional context, enhancing the information available for debugging.
- Leverage
- Specific Error Checks:
- Check for specific error types using type assertions or switches to handle different error scenarios appropriately.
- Transparent APIs:
- Design APIs with explicit error values, avoiding shadowing of errors, and providing clear documentation for users.
- Balancing Verbosity and Clarity:
- Include relevant context in error messages without unnecessary verbosity, striking a balance between informative and concise messages.
B. Encouragement for Adopting Best Practices
Adopting best practices in error handling not only aligns with Go’s design philosophy but also contributes to the development of more maintainable and resilient code. Encouragements for developers include:
- Consistency Across Codebase:
- Maintain consistency in error handling practices across the codebase to enhance readability and streamline debugging efforts.
- Continuous Learning:
- Stay informed about best practices by studying real-world examples, exploring popular libraries, and learning from the Go community.
- Feedback Loop:
- Establish a feedback loop for error handling, encouraging code reviews that focus on error scenarios to identify improvements and ensure adherence to best practices.
C. The Role of Effective Error Handling in Building Robust Go Applications
Effective error handling plays a pivotal role in building robust and resilient Go applications. It contributes to:
- Reliability:
- Reliable applications handle errors gracefully, preventing unexpected crashes and providing a smoother user experience.
- Debuggability:
- Clear error messages and proper error wrapping facilitate debugging, allowing developers to quickly identify and resolve issues.
- Maintainability:
- Well-structured error handling promotes code maintainability by making it easier for developers to understand and modify error-related logic.
- User Experience:
- Thoughtful error handling contributes to a positive user experience by anticipating and gracefully handling potential issues.
In conclusion, mastering error handling in Go is not just about addressing failures; it’s about creating a resilient and user-friendly software ecosystem. By embracing best practices, developers contribute to the success of their projects and foster a culture of reliability and excellence in the Go community.